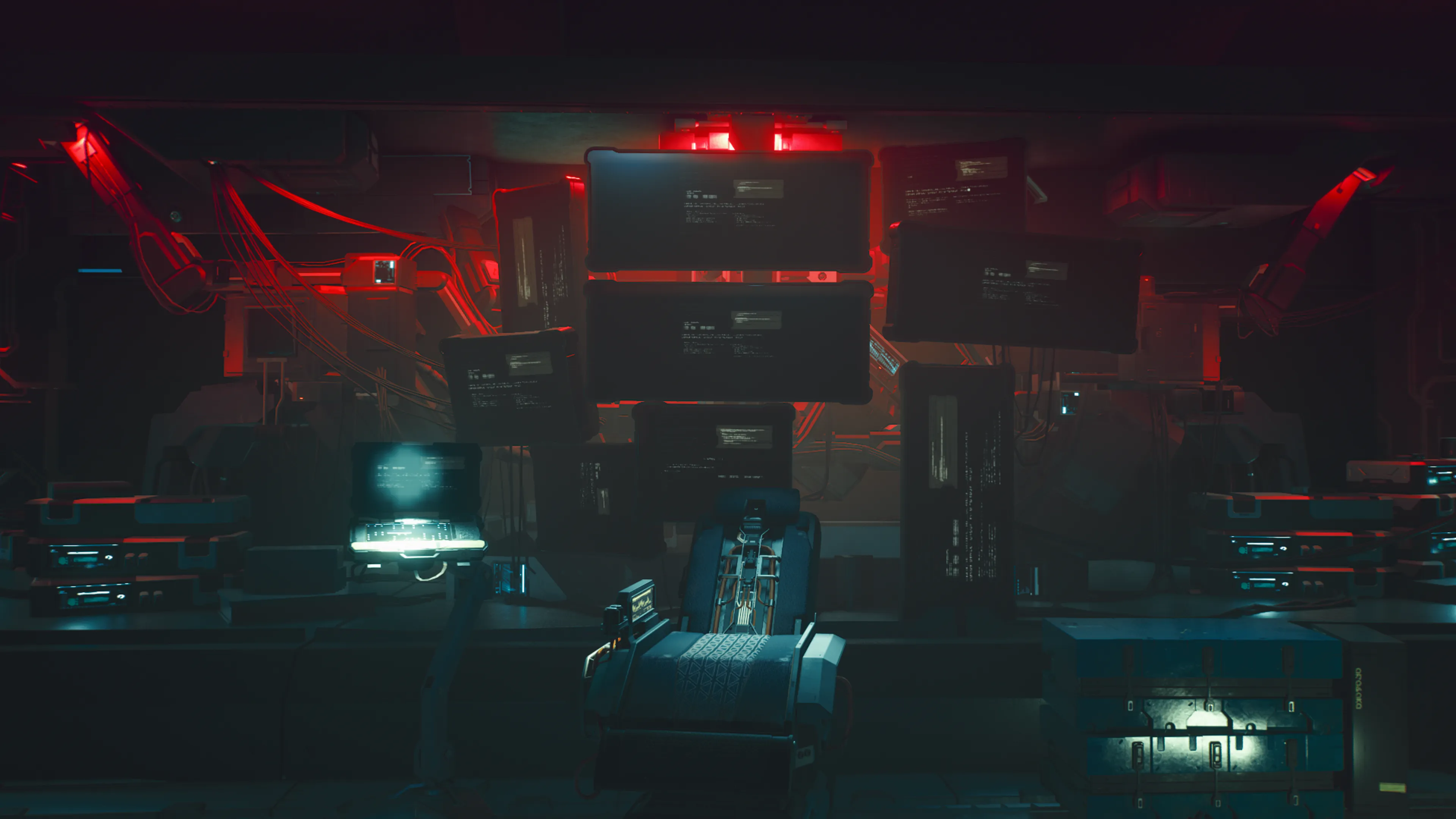
Exception Handling in Python: A Detailed Guide
Exception handling is a crucial aspect of writing robust and error-free code in any programming language, including Python. In this blog post, we’ll explore how to handle exceptions effectively, using a simple example of calculating the average of a list of numbers. We’ll walk through the process of identifying potential issues, implementing error handling, and even creating custom exceptions for more specific error messages.
Calculating the Average: A Simple Example
Let’s start with a straightforward task: calculating the average of a list of numbers. Here’s a basic implementation of the function to achieve this:
def calculate_average(numbers):
total = sum(numbers)
average = total / len(numbers)
return average
numbers = [10, 20]
average = calculate_average(numbers)
print(f"The average is {average}")
When we run this code, we get the following output:
The average is 15.0
Handling Edge Cases
Now, let’s consider an edge case: what if the list is empty?
def calculate_average(numbers):
total = sum(numbers)
average = total / len(numbers)
return average
numbers = []
average = calculate_average(numbers)
print(f"The average is {average}")
Running this code results in an error:
Traceback (most recent call last):
File "sample.py", line 9, in <module>
average = calculate_average(numbers)
^^^^^^^^^^^^^^^^^^^^^^^^^^
File "sample.py", line 3, in calculate_average
average = total / len(numbers)
~~~~~~^~~~~~~~~~~~~~
ZeroDivisionError: division by zero
Improving Error Messages
The error occurs because the list is empty, leading to a ZeroDivisionError
. However, the error message is not very descriptive. To make it clearer, we can add a conditional check and raise a more specific ValueError
:
def calculate_average(numbers):
total = sum(numbers)
if len(numbers) == 0:
raise ValueError("Cannot calculate average of an empty list")
average = total / len(numbers)
return average
numbers = []
average = calculate_average(numbers)
print(f"The average is {average}")
This time, running the code results in:
Traceback (most recent call last):
File "sample.py", line 11, in <module>
average = calculate_average(numbers)
^^^^^^^^^^^^^^^^^^^^^^^^^^
File "sample.py", line 4, in calculate_average
raise ValueError("Cannot calculate average of an empty list")
ValueError: Cannot calculate average of an empty list
While the error message is now more descriptive, the program still terminates before finishing execution. To handle this properly, we need to use try
and except
blocks.
Using Try-Except Blocks
We need to wrap the function call in a try
block and handle the ValueError
in an except
block:
def calculate_average(numbers):
total = sum(numbers)
if len(numbers) == 0:
raise ValueError("Cannot calculate average of an empty list")
average = total / len(numbers)
return average
numbers = []
try:
average = calculate_average(numbers)
print(f"The average is: {average}")
except ValueError as e:
print(f"Error: {e}")
Running this code results in:
Error: Cannot calculate average of an empty list
Creating Custom Exceptions
For more specific error handling, we can create a custom exception class. Let’s define an EmptyListError
that inherits from the Exception
class:
class EmptyListError(Exception):
def __init__(self, list_size=0):
self.list_size = list_size
message = f"Cannot calculate average of an empty list (size: {list_size})"
super().__init__(message)
def calculate_average(numbers):
total = sum(numbers)
if len(numbers) == 0:
raise EmptyListError(len(numbers))
average = total / len(numbers)
return average
numbers = []
try:
average = calculate_average(numbers)
print(f"The average is: {average}")
except EmptyListError as e:
print(f"Error: {e}")
Running this updated code gives us:
Error: Cannot calculate average of an empty list (size: 0)
Further Considerations
While we have addressed the issue of an empty list, there are other edge cases to consider, such as lists containing non-numeric values. Handling these scenarios involves more advanced techniques, such as multiple exception handling, exception chaining, and custom error messages.
Conclusion
Effective exception handling is a key skill for writing reliable Python code. By understanding how to identify and manage potential errors, you can create programs that handle unexpected situations gracefully. Always remember that there’s room for improvement, and exploring more advanced exception handling techniques can further enhance the robustness of your code.
Thank you for reading, and happy coding!